Selenium Python is a powerful combination of two popular tools: Selenium WebDriver and the Python programming language. Together, they allow you to automate web testing tasks, such as logging in to websites, filling out forms, and clicking buttons.
Web automation testing can save you a lot of time and effort, especially if you have to test your website on multiple browsers and devices. Selenium in Python makes it easy to write test scripts that run on any browser or device that Selenium supports.
In this blog post, we will show you how to use Selenium Automation Testing using Python. We will cover the basics of Selenium WebDriver, as well as how to write test scripts in Python. We will also provide some tips and best practices for Selenium testing.
Contents
Getting Started With Selenium Python Automation
Selenium Python is a powerful combination of Selenium WebDriver and Python that allows you to automate web testing tasks, such as logging in to websites, filling out forms, and clicking buttons. It is a popular choice for web automation testing because it is easy to learn and use, cross-browser and cross-platform, and open source and free to use.
To get started with Selenium in Python automation, you will need to install the following prerequisites:
- Python
- Selenium WebDriver
- A web browser driver (e.g., ChromeDriver, FirefoxDriver)
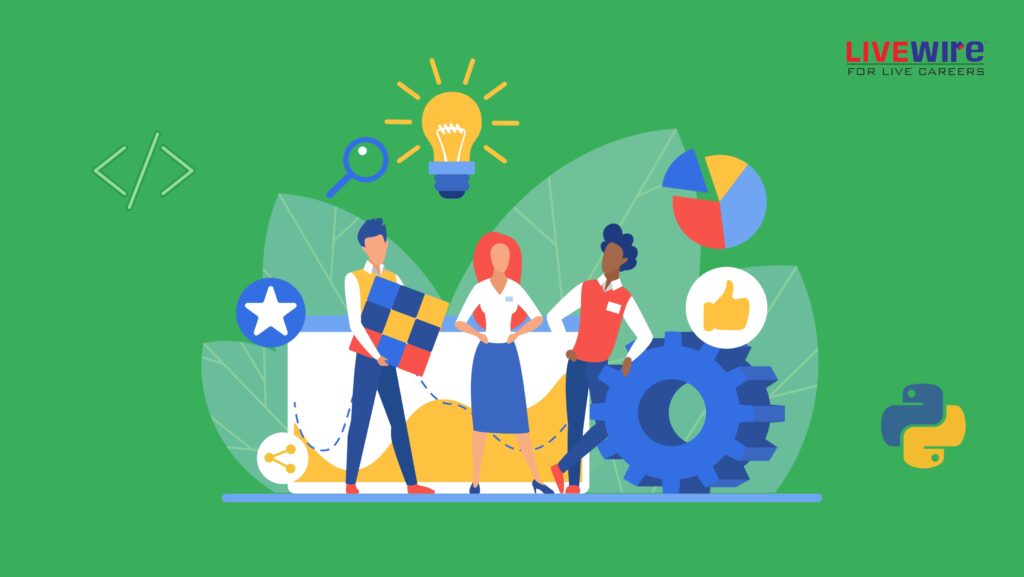
Once you have installed the prerequisites, you can start writing Selenium Using Python test scripts. A typical Selenium-Python test script will follow these steps:
- Import the necessary Selenium libraries.
- Create a WebDriver instance.
- Navigate to the website you want to test.
- Find the web components you wish to work with.
- Perform actions on the web elements (e.g., click buttons, fill out forms).
- Verify the results of the actions.
Close the WebDriver instance.
Here is a simple example of a Selenium Python test script:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.google.com")
search_input = driver.find_element_by_name("q")
search_input.send_keys("Selenium Python")
search_input.submit()
search_results = driver.find_elements_by_class_name("r")
assert len(search_results) > 0
driver.close()
This test script will open the Google homepage, enter the search term “Selenium Python”, and click the Search button. It will then verify that there is at least one search result.
You can use Selenium-Python to write test scripts for a variety of web testing tasks, such as Functional testing, Regression testing, Performance testing, and Cross-browser testing. If you are interested in learning more about Selenium-Python automation, there are a number of resources available in training centres, online and in libraries. You can also find many tutorials and examples on the Selenium website.
What Is Selenium In Python?
Selenium Is A Free, Open-Source Automation Testing Framework That Allows Testers To Control Web Browsers Using Programming Languages Like Python. It Supports A Wide Range Of Browsers And Operating Systems And Is One Of The Most Popular Web Automation Tools Available.
Selenium consists of two main components:
- Selenium WebDriver: This is the core component of Selenium and provides the functionality for interacting with web browsers.
- Selenium IDE: This is a graphical user interface (GUI) that allows testers to record and playback their interactions with web browsers.
Selenium also used to automate a wide range of web testing tasks, such as:
- Functional testing: This involves testing the functionality of a web application to ensure that it works as expected.
- Regression testing: This involves retesting previously tested functionality to ensure that it is not broken by new changes.
- Integration testing: This involves testing how different components of a web application work together.
- User acceptance testing (UAT): This involves testing a web application with real users to ensure that it meets their needs.
There are many benefits to using Selenium-Python, including:
- It is open-source and free.
- It works with a broad variety of operating systems and browsers.
- It is simple to use and learn.
- It is very versatile.
- It has a huge and thriving user and contributor community.
Binding Selenium With Python
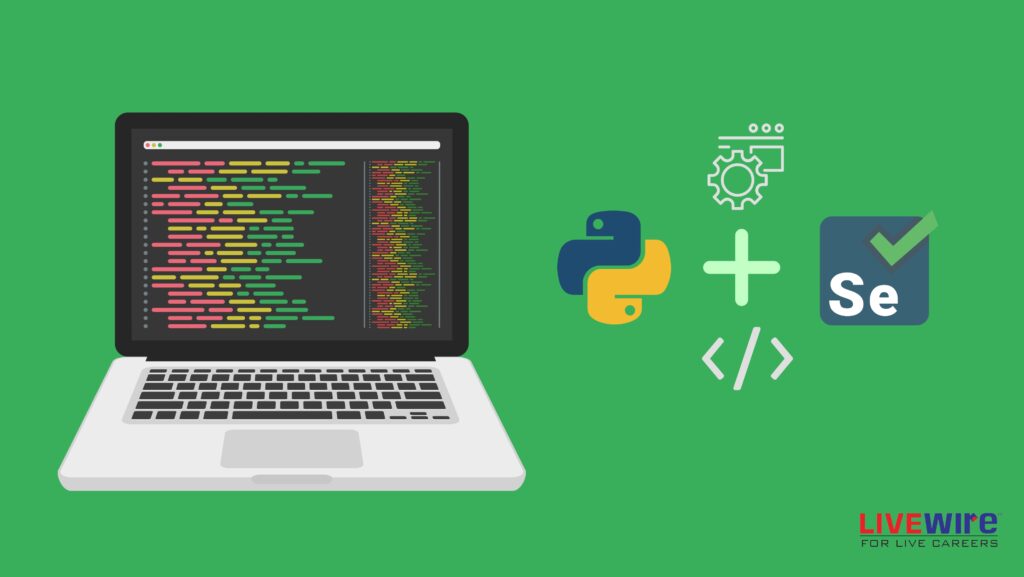
Selenium is a popular web automation framework used to automate browsers and test web applications. It is an open-source tool that is supported by a large community of developers. Numerous tasks may automate with Selenium, including:
- Opening and closing browsers
- Navigating to web pages
- Clicking buttons and links
- Entering text into fields
- Selecting items from drop-down menus
- Verifying the contents of web pages
Selenium used with a variety of programming languages, including Python. Binding Selenium with Python allows developers to write test scripts in a concise and easy-to-read way.
To bind Selenium in Python, you need to install the selenium package. You can do this using pip:
pip install selenium
Once you have installed the selenium package, you can start writing test scripts. Here is a simple example of a test script that uses Selenium to open a web browser and navigate to a web page:
from selenium import webdriver
# Create a new WebDriver instance
driver = webdriver.Chrome()
# Navigate to the Google homepage
driver.get("https://www.google.com")
# Close the browser
driver.quit()
This script will open a Chrome browser and navigate to the Google homepage. It will then close the browser after a few seconds.
You can use Selenium to automate a wide range of tasks, such as:
- Testing a web application’s functionality
- Filling out forms
- Scraping data from web pages
- Performing regression testing
Binding Selenium with Python is a great way to automate web browsers and test web applications. It is a powerful and flexible tool that may used to automate a wide range of tasks.
Web Automation Using Python And Selenium
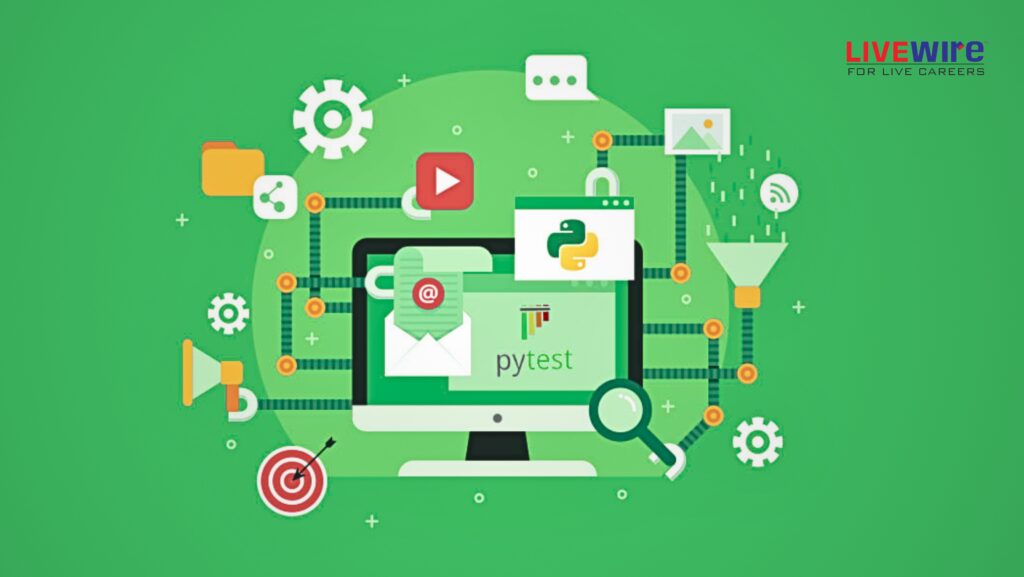
Web automation is the process of using software to automate tasks that are typically performed manually by humans on a web browser. This can include tasks such as logging in to websites, filling out forms, clicking buttons, and extracting data.
Python is a general-purpose programming language that is well-suited for web automation. It has an active community of users and developers and is simple to learn. A framework for web automation called Selenium offers a number of tools for interfacing with web browsers. Python is one of the many programming languages supported by Selenium.
Benefits of Web Automation
There are many benefits to using web automation, including:
- Increased efficiency and productivity
- Reduced errors
- Improved consistency
- Scalability
- Reduced costs
Web Automation with Python and Selenium
To automate web tasks with Python and Selenium, you will need to:
- Install Python and Selenium.
- Choose a web driver. A web driver is a tool that allows Selenium to interact with web browsers. The most popular web driver for Python is ChromeDriver.
- Write a Selenium script. A Selenium script is a program that uses Selenium to automate web tasks. Selenium scripts can be written in any programming language that Selenium supports, but Python is a good choice for beginners.
- Run the Selenium script.
Web Automation Using Python and Selenium can be used for a variety of tasks, including Web testing, Web scraping and Task automation.
Learn Web Scraping Using Selenium Python
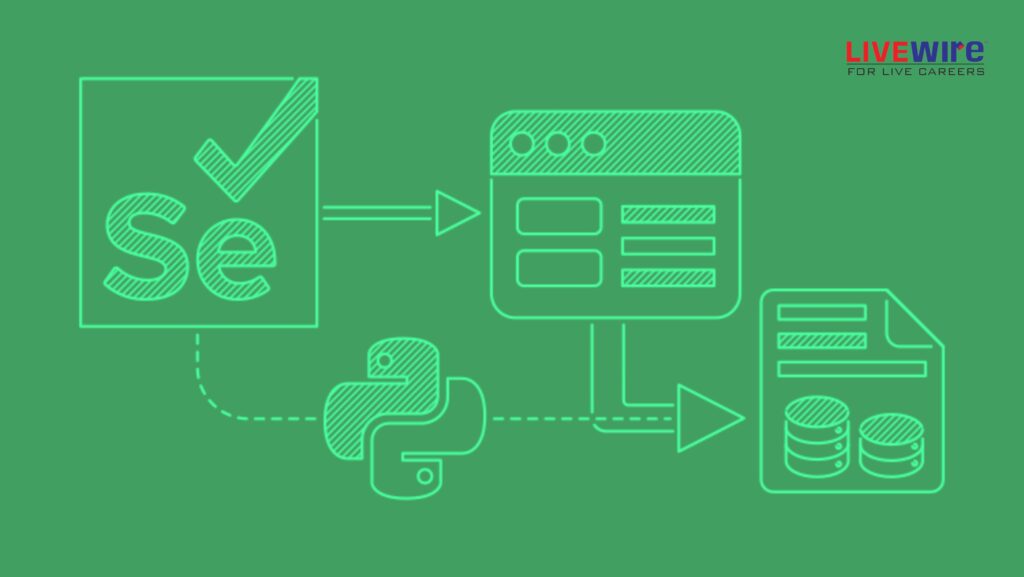
The process for gathering data from web pages is called web scraping. It is a strong tool with a wide range of uses, including market research, data mining, and application development.
Selenium is one of the most widely used technologies for web scraping. Selenium is a web automation framework that enables you to programmatically control a web browser. This makes it ideal for scraping websites, as you can use Selenium to simulate the actions of a human user, such as clicking buttons, filling out forms, and scrolling through pages.
To learn web scraping using Selenium Python, you will need to:
- Install Selenium and the corresponding WebDriver for your browser.
- Import the necessary libraries into your Python code.
- Create a new WebDriver instance and navigate to the website you want to scrape.
- Locate the elements on the page that contain the data you want to extract.
- Extract the data from the elements and store it in a variable or data structure.
- Close the WebDriver instance.
Once you have extracted the data from the website, you can store it in a database, spreadsheet, or other data structure. You can then use the data for your own purposes, such as building an application or creating a report.
Web scraping is a strong technique that may be applied to many different situations. You may access a variety of information that can be utilized to advance your professional, academic, or personal endeavours by learning Selenium-Python web scraping.
How To Test Selenium Python Framework?
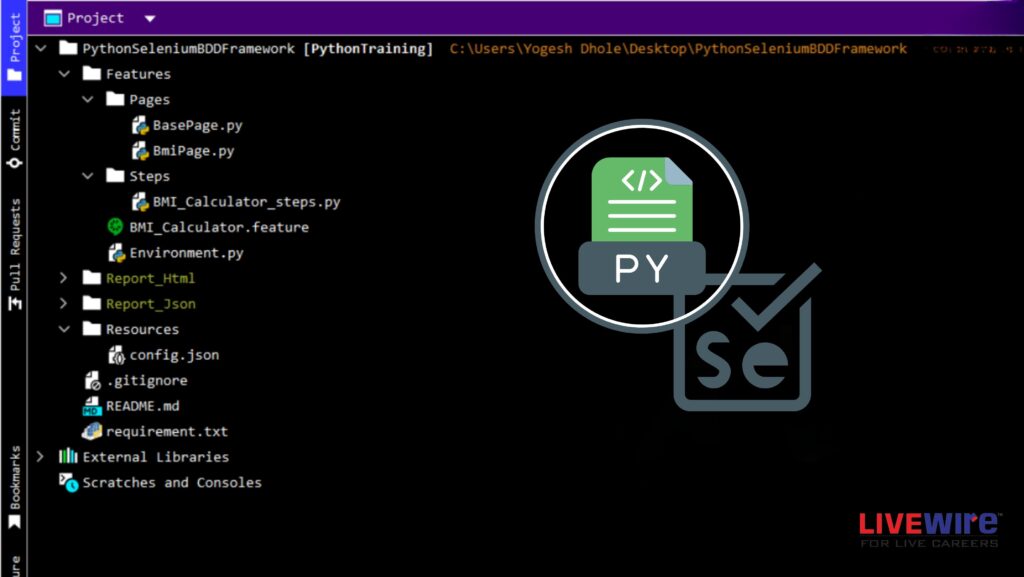
Testing a Selenium Python framework is essential to ensure that your automated tests are reliable and effective. The best approach will depend on your specific needs and requirements, but some common testing approaches include:
- Unit testing: Unit testing frameworks allow you to test individual units of code, such as functions or classes, in isolation. This can help you to identify and fix bugs early on.
- Browser testing: Selenium Web Browser testing frameworks allow Automation tests that interact with a web browser. This can be useful for testing the overall functionality of your framework, as well as specific features of your web application.
- Continuous integration (CI): A CI server can automatically run your tests whenever you make changes to your code, helping you to catch bugs quickly.
- Test management tools:Test management tools can help you track your progress and generate reports, making it easier to manage your testing process.
Which testing approach is best for you will depend on your specific needs and requirements. However, it is important to have a comprehensive testing plan in place to ensure that your Selenium-Python framework is reliable and effective.
Here are some additional tips for testing a Selenium-Python framework:
- Use a variety of test data. This will help you to ensure that your framework is able to handle a wide range of inputs and outputs.
- Log your tests. This will assist you in resolving any issues that may arise.
- Review your test results carefully. This will help you to identify any areas where your framework needs to be improved.
By following these tips, you can ensure that your Selenium-Python framework is thoroughly tested and reliable. If you want to Learn Selenium with Python Course, you can check our course details here.
FAQs
With its increased usage, integration with AI and ML, support for current web technologies, improved performance testing, and growing importance in mobile testing, Selenium with Python has a promising future.
Python has better support for Selenium than Ruby. There are more Python libraries and frameworks available for Selenium, and the Python community is more active in developing and maintaining Selenium tools.
Python is the best and easiest language to learn for test automation with Selenium. It is a general-purpose language with a simple syntax, making it easy to learn and use. Python also has a large community of developers who contribute to the Selenium ecosystem, so there are many resources available to learn Selenium with Python.
The most popular Python framework for Selenium is PyTest. PyTest is a simple and easy-to-use framework that provides a variety of features for writing and executing test cases. Other popular Python frameworks for Selenium include unittest, Robot Framework, and Serenity BDD.
Both Python and Java are in demand for Selenium automation, but Python is becoming increasingly popular. This is due to Python’s simplicity, ease of use, and large community of developers. Overall, Python is a great choice for Selenium automation. It is a popular and well-supported language for with a bright future.